Understanding a Specific Programming Language
This document explores a programming language known for its use in developing applications. Its features, applications, and impact on software development are examined. The language's design principles and role in the broader software development landscape are discussed.
This particular language is frequently used to create applications with specialized functionality. It offers a range of features for developers, including robust frameworks and a large community. Applications built using this language can span various domains, demonstrating its versatility.
This language has influenced the development of later programming technologies. Its historical context and evolution provide insights into software development trends over time.
This exploration will proceed to analyze the technical aspects of the language, including its syntax, data structures, and object-oriented methodologies.
What is Java
Understanding Java involves grasping its fundamental characteristics. These essential aspects are crucial for comprehending the language's utility and role in software development.
- Object-Oriented
- Platform-Independent
- Robust
- Secure
- Versatile
- Extensive Libraries
- Large Community
Java's object-oriented nature fosters modularity and reusability. Its platform-independent characteristic allows code to run on various systems, reducing development time. Robustness minimizes errors, while security safeguards against malicious attacks. Versatility allows applications in diverse fields. Extensive libraries provide pre-built tools for developers. A large, active community offers support and resources. These aspects collectively define Java's position as a powerful and enduring programming language. For instance, applications like Android apps and enterprise software frequently employ Java's robust and versatile nature, demonstrating its suitability for complex projects.
1. Object-Oriented
Java's object-oriented nature is a defining characteristic. This paradigm significantly impacts the language's structure, design, and application. Core principles like encapsulation, inheritance, and polymorphism directly influence how programs are constructed in Java. Encapsulation bundles data and methods that operate on that data within a class, promoting organization and data integrity. Inheritance allows the creation of new classes (subclasses) based on existing ones (superclasses), facilitating code reuse and establishing a hierarchical relationship between classes. Polymorphism enables objects of different classes to be treated as objects of a common type, enhancing flexibility and extensibility. These elements, fundamental to object-oriented programming, contribute to building robust, scalable, and maintainable applications in Java. Consider a software system managing customer accounts. Using an object-oriented approach, each customer can be represented as an object, encapsulating data like name, address, and account balance. Inheritance might be employed to create subclasses like "Premium Customer" or "Corporate Customer," adding specific functionalities to these customer types. Polymorphism allows uniform treatment of all customer objects, regardless of their type. This structured, object-oriented approach makes the system more adaptable and manageable as needs evolve.
The object-oriented design of Java facilitates easier maintenance and updates, as modifications to one part of the system are less likely to disrupt other parts due to the encapsulated nature of classes. This principle is crucial for large-scale projects where codebase complexity is high. Applications requiring flexibility and scalability, such as banking software or complex data processing systems, greatly benefit from the object-oriented structure of Java. By employing these principles, developers can create programs that are more adaptable to future demands. The design structure itself encourages clean separation of concerns, improving code organization and reducing errors.
In summary, the object-oriented approach intrinsic to Java is pivotal. Its emphasis on classes, objects, and relationships directly affects program architecture and maintainability. The organizational and modular benefits inherent in this design style, along with features like encapsulation and inheritance, are crucial elements in building complex software systems effectively and efficiently. The structured approach offers robustness and allows for future scalability, making Java well-suited for various development projects, from smaller-scale applications to enterprise-level systems.
2. Platform-Independent
A key aspect of Java is its platform independence. This characteristic distinguishes Java from many other programming languages, influencing its widespread adoption and applications.
- Bytecode Execution
Java code is compiled into bytecode, an intermediate form. This bytecode is not directly executed by the hardware but by a Java Virtual Machine (JVM). The JVM, platform-specific software, interprets the bytecode and executes it on the target system. This crucial design allows the same Java bytecode to run on different operating systems (Windows, macOS, Linux) without modification. This contrasts sharply with languages that require separate compilation and linking for each platform. The platform independence reduces the need for separate versions of the software and simplifies deployment across diverse environments.
- Portability of Applications
The platform independence of Java facilitates the portability of applications. A software application written in Java can theoretically run on any system with a compatible JVM. This portability can be a significant advantage for businesses seeking a unified platform for development and deployment across a variety of computing environments. It dramatically reduces the effort and costs associated with maintaining separate versions of the software for different operating systems.
- Reduced Development Time and Costs
Developers can focus on creating the application logic without extensive concern for the underlying platform. This platform independence streamlines development by reducing the time and resources necessary for adapting code to various operating systems. Furthermore, it minimizes the risk of errors arising from platform-specific dependencies.
- Wider Reach and Versatility
The ability to deploy applications across platforms significantly broadens Java's reach. It permits the same codebase to serve diverse end-user needs. This translates to significant potential for widespread adoption and utilization in different markets.
In essence, Java's platform independence is a significant strength. By abstracting the platform from the code, Java promotes efficient development, simplifies deployment, and enhances the portability of applications. This feature profoundly impacts the use and effectiveness of Java in various contexts, from small-scale software to intricate enterprise applications.
3. Robust
Robustness in Java is a critical attribute, directly impacting the reliability and stability of applications. It stems from design choices that emphasize error prevention and handling, security, and resilience. This characteristic ensures applications endure unforeseen circumstances and remain functional under stress.
- Exception Handling
Java's robust exception-handling mechanism allows programs to gracefully manage and recover from errors. This means that if an unexpected problem arises (e.g., a file not found, a network interruption), the application doesn't crash. Instead, it can handle the situation appropriately, perhaps logging the error, prompting the user, or taking corrective actions. This proactive approach minimizes the risk of application failures and enhances user experience by preventing abrupt terminations.
- Type Safety
Java's strong type system helps prevent errors during compilation. The explicit declarations of data types allow the compiler to identify potential type-mismatch issues early on. This reduces the occurrence of runtime errors, which are a significant source of instability in applications. The stringent type system contributes to the robustness by ensuring that data is used and manipulated according to its intended purpose.
- Memory Management
Java's automatic garbage collection mechanism manages memory automatically. This significantly reduces memory leaks and other memory-related problems, making applications more resilient. The automatic memory management approach, freeing developers from manual memory management tasks, enhances the stability and longevity of programs. This automated system reduces the possibility of critical errors related to memory allocation and deallocation, a frequent source of application instability in other languages.
- Security Features
Java incorporates security mechanisms to protect applications from various threats. Features like secure access controls and restricted code execution prevent unauthorized access and malicious code execution. This level of security within the language's design directly contributes to application robustness by mitigating the potential impact of security breaches.
These features of robustness in Java contribute to its capacity for building dependable and secure applications. The careful consideration of error handling, type safety, memory management, and security safeguards is fundamental to ensuring long-term viability and reliability of Java-based systems. Robustness, thus, is not merely a feature but a fundamental aspect of Java's design philosophy, ensuring applications can handle various conditions and endure over time.
4. Secure
Security is a crucial component of Java's design. The language's inherent security features contribute significantly to the reliability and trustworthiness of applications developed using it. Java's security model is multifaceted, addressing various potential vulnerabilities. A core aspect involves the use of bytecode and the Java Virtual Machine (JVM). Bytecode, the intermediate form of Java code, is verified by the JVM before execution. This verification process helps mitigate the risk of malicious code execution. Furthermore, Java's security model employs access control mechanisms, restricting access to resources based on the permissions assigned to the code. This approach prevents unauthorized access to critical data or functions, thus guarding against security breaches.
Real-world applications demonstrate the importance of security in Java. Financial institutions, for example, frequently utilize Java for their critical systems, such as online banking platforms. The inherent security features of Java are essential in safeguarding sensitive financial data and preventing fraudulent activities. Similarly, government agencies often leverage Java for applications handling sensitive information, highlighting the crucial role of security in ensuring data integrity and protection from cyberattacks. The security mechanisms integrated into the language, from sandboxing to code verification, directly contribute to the applications' trustworthiness in these contexts. This is critical for preventing data breaches and protecting valuable information. The security features contribute to the stability and reliability of systems. This is reflected in the sustained use of Java in critical systems.
In conclusion, security is an integral aspect of the Java programming language. The language's design incorporates features that address various potential security threats, ultimately contributing to the reliability and trustworthiness of Java-based applications. The use of bytecode verification, access control, and other security mechanisms ensures that sensitive data and resources are protected. The ability of Java to perform securely is especially critical for high-stakes applications and systems where data integrity is paramount.
5. Versatile
Java's versatility is a significant factor in its widespread adoption and enduring utility. This adaptability allows the language to be applied across diverse domains, from mobile applications to enterprise-level systems. This section explores the specific facets of this adaptability and demonstrates how they contribute to Java's overall utility.
- Extensive Libraries and Frameworks
Java boasts a vast collection of libraries and frameworks. These pre-built components simplify development by providing ready-made solutions for common tasks. For instance, libraries like Swing and JavaFX aid in graphical user interface (GUI) development. Frameworks like Spring and Hibernate streamline enterprise applications, handling complex aspects such as database interaction and application structure. This readily available support drastically reduces development time and effort, making development more efficient and faster, thereby promoting widespread usability.
- Platform Independence
Java's platform-independent nature further enhances its versatility. Written code can run on various operating systems without modification, expanding the reach and applicability of Java-based applications. This characteristic makes Java suitable for diverse environments, from embedded systems to desktop computers, ensuring consistent performance across different hardware and software platforms. This portability allows for faster and more cost-effective deployment across diverse environments.
- Object-Oriented Structure
Java's object-oriented design facilitates modularity and code reuse. This structure makes it easier to build complex applications by breaking them down into smaller, manageable components. The organized structure of classes and objects allows for flexible and scalable development. This promotes maintainability and reusability, critical for long-term projects and large-scale software development efforts. Object-oriented programming principles significantly enhance the language's adaptability to different project requirements.
- Support for Diverse Programming Styles
While object-oriented programming is a mainstay, Java also accommodates other programming paradigms to a degree. Developers can use imperative approaches alongside object-oriented elements. This flexibility allows for a diverse range of coding approaches and styles, enhancing developers' ability to employ the most effective methods for given tasks. This adaptability is especially valuable for integrating diverse coding approaches to existing project elements. The flexibility in programming styles makes it suitable for integration with existing applications and diverse project methodologies.
Java's versatility emerges from a combination of its extensive libraries, platform independence, robust object-oriented structure, and adaptability to different programming styles. This multifaceted nature makes it a powerful and versatile tool applicable across diverse sectors, from mobile development and data processing to enterprise solutions. This flexibility ensures its relevance in rapidly evolving technological landscapes.
6. Extensive Libraries
Extensive libraries are a defining characteristic of Java. These pre-built components significantly influence the language's utility and application in various domains. The availability of a rich ecosystem of libraries reduces development time, facilitates complex tasks, and contributes to the overall strength and versatility of Java-based projects.
- GUI Development
Libraries like Swing and JavaFX provide tools for creating graphical user interfaces (GUIs). This aspect is crucial for user-friendly applications. GUI development in Java is facilitated by these readily available libraries, empowering developers to focus on application logic rather than low-level graphical elements. This characteristic is apparent in desktop applications, mobile interfaces built using Java, and intricate graphical elements in enterprise software.
- Database Interaction
Libraries like JDBC (Java Database Connectivity) provide a standard way for Java programs to interact with various database systems. This ensures consistency and portability. Developers can readily access and manipulate data in diverse databases without having to create customized solutions. This facilitates integration with SQL databases, ensuring streamlined data management within applications and streamlining data manipulation tasks.
- Network Programming
Java's network libraries offer robust support for networking functionalities. Libraries for tasks such as socket programming, HTTP requests, and XML processing are fundamental for building network-centric applications. The availability of these libraries allows the development of client-server applications, web services, and network utilities quickly and efficiently. This enables rapid development of applications requiring communication and data exchange across networks.
- Security Implementations
Several libraries support securing applications. These libraries handle various security tasks, from encryption to digital signatures. Security libraries contribute to building secure applications by providing comprehensive solutions for data protection and access controls. This is essential in applications handling sensitive information, such as financial transactions or secure communication systems.
The extensive library support in Java is crucial for its versatility and efficiency. The availability of readily usable code for common tasks accelerates the development process and significantly reduces the need for redundant code creation, allowing developers to concentrate on core application logic and innovation. This efficiency is crucial for creating high-quality applications across a broad range of domains.
7. Large Community
A significant aspect of Java's enduring relevance is its large and active community. This community fosters ongoing development, support, and resource sharing. The size and dedication of the Java community directly impact the language's continued evolution and utility. A robust community contributes to a thriving ecosystem of libraries, frameworks, and tools, enhancing Java's versatility and applicability. This support network is particularly beneficial for developers encountering challenges or seeking solutions to specific problems within Java's extensive codebase.
The community's contributions are multifaceted. Active forum discussions and comprehensive documentation address diverse use cases, providing valuable resources for developers. Open-source projects developed and maintained by community members extend Java's capabilities, providing innovative solutions for specific tasks. This collaborative nature enhances the language's robustness and adaptability. The shared knowledge and continuous improvement facilitated by this community empower developers to address complex issues, potentially leading to the creation of innovative applications. Examples include advancements in enterprise solutions, mobile application development, and the ongoing support for legacy systems. The constant evolution of the Java community is crucial for maintaining its relevance in a rapidly changing technology landscape. This ongoing contribution ensures the maintenance of existing frameworks and facilitates the development of new ones.
The large, active Java community, therefore, is a critical component of what makes Java a valuable programming language. This community fosters the ongoing evolution of the language and its tools, maintaining its usefulness and applicability in diverse contexts. The interconnectedness and shared resources of the community prove fundamental to Java's sustained relevance and continued adoption. The practical implications of a large and engaged developer community are clear in the constant updates, readily available solutions, and innovations arising within the Java ecosystem. This ecosystem, empowered by a strong community, remains a vital force in software development.
Frequently Asked Questions about Java
This section addresses common inquiries regarding the Java programming language. These questions aim to clarify key concepts and dispel potential misconceptions.
Question 1: What is Java, and why is it used?
Java is a high-level, object-oriented programming language known for its platform independence. Applications written in Java can run on various operating systems without recompilation, thanks to the Java Virtual Machine (JVM). This platform independence contributes to its widespread use in diverse applications, from enterprise software to mobile development.
Question 2: How does Java differ from other programming languages like Python or C++?
Java is object-oriented, requiring code to be organized around objects. Python, by contrast, is more flexible in its approach and permits a variety of programming paradigms. C++ offers greater control over system resources but comes with more complexity. Java's platform independence is a distinguishing feature, allowing programs to run on different systems without modification.
Question 3: What are the advantages of using Java for development?
Java offers several advantages, including platform independence, robustness, and extensive libraries. Its security features contribute to the reliability of applications, while its large community ensures ongoing support and resources. Furthermore, Java's extensive libraries streamline development by providing pre-built components.
Question 4: What is the Java Virtual Machine (JVM), and why is it important?
The JVM is a crucial component of Java's architecture. It acts as an intermediary between the Java bytecode and the underlying operating system. This intermediary allows Java programs to run on different platforms without modification, contributing to Java's platform-independence characteristic.
Question 5: What are some common applications developed using Java?
Java's versatility is demonstrated in diverse application areas. It is used in enterprise applications, mobile development (Android), big data processing, and web applications. Its use extends to scientific computing, embedded systems, and other specialized domains.
Understanding these key aspects provides a foundation for comprehending Java's unique position in software development.
This concludes the FAQ section. The next section will delve into the specifics of Java development, exploring topics like syntax, data structures, and common libraries.
Conclusion
This exploration of the programming language, often referred to as Java, has underscored its multifaceted nature. Key characteristics, including its object-oriented structure, platform independence, robustness, extensive libraries, and the substantial support of a large community, have been examined. The language's versatility, allowing development across diverse domains, has been highlighted. The discussion has demonstrated how these features contribute to Java's enduring relevance and utility in contemporary software development.
The evolution of Java reflects the dynamic nature of software development. Its enduring presence signifies a commitment to the principles of modularity, security, and platform-independence. Future advancements in this language are likely to build upon these established strengths. The substantial community support and the continued development of libraries provide a strong foundation for innovation and ongoing evolution in the field of software development. Developers seeking a robust, versatile, and well-supported language for their projects will find Java to be a powerful choice. This programming language's potential and impact across diverse software applications will continue to be a vital consideration in the world of technology.
Article Recommendations
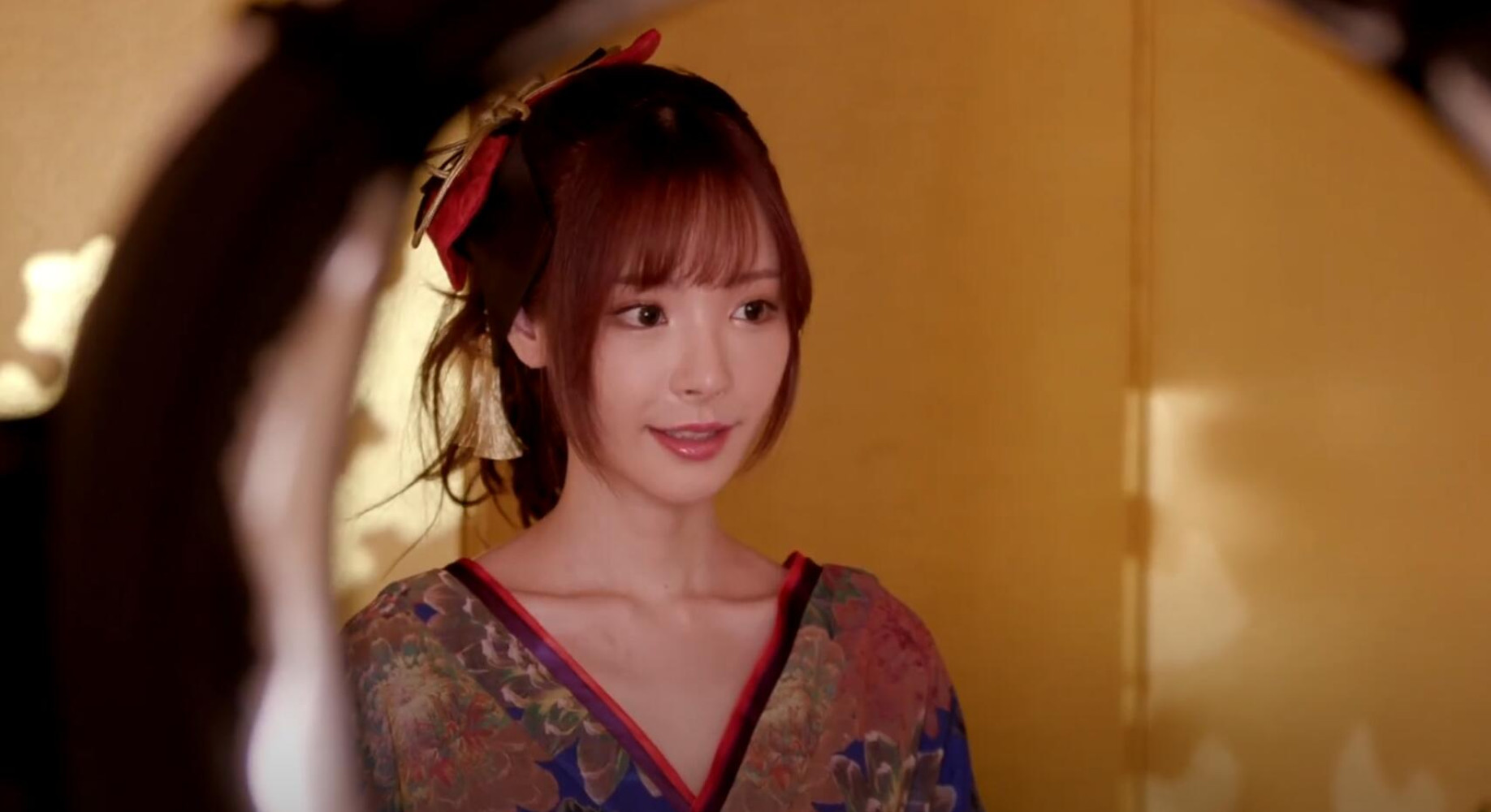
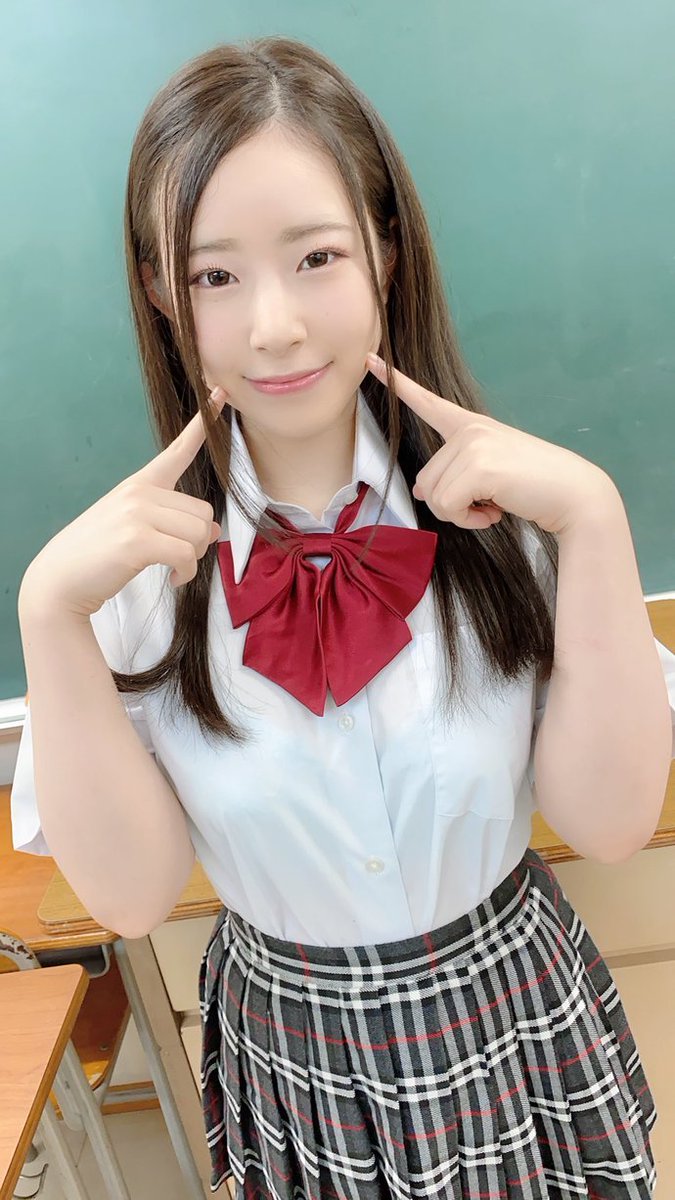
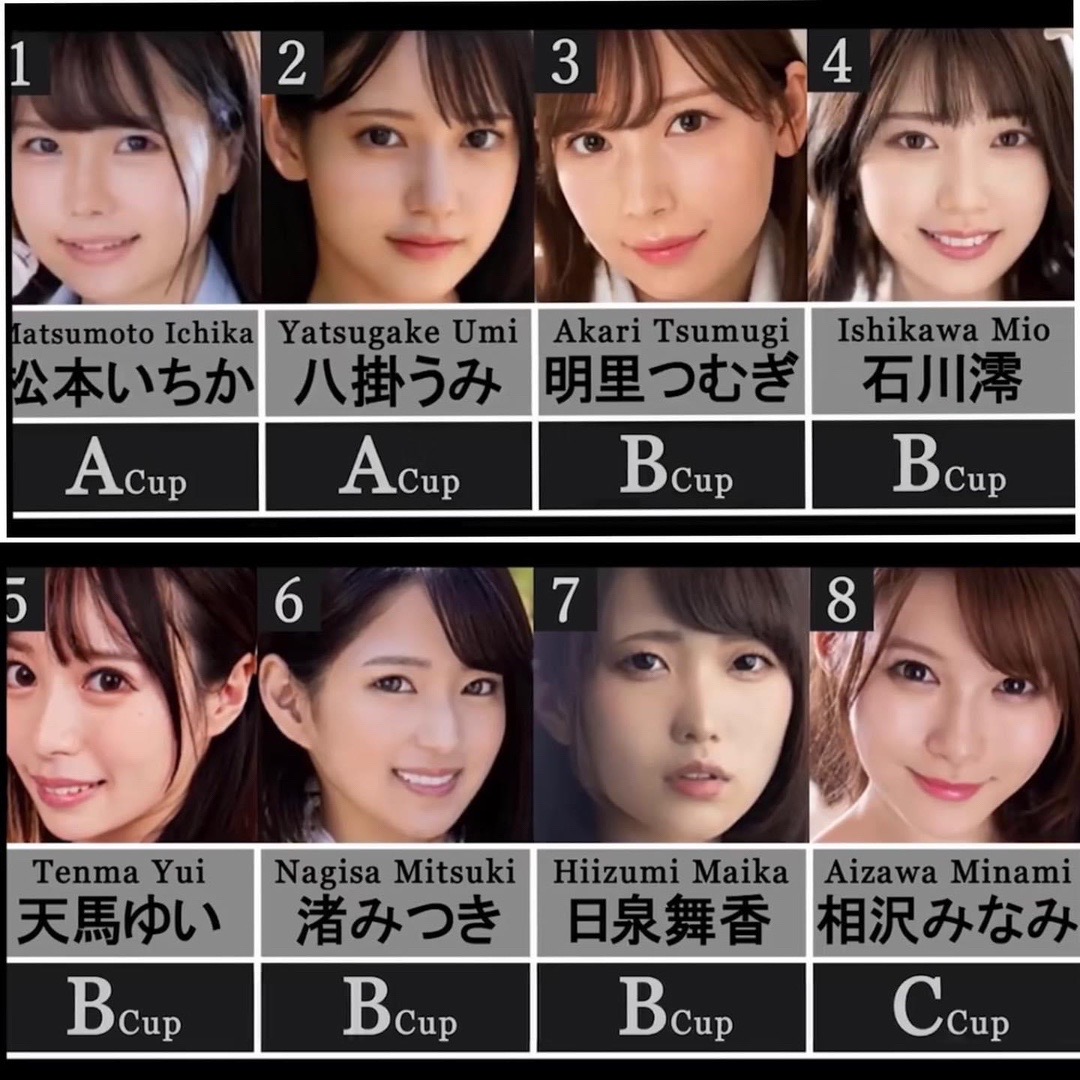
ncG1vNJzZmibkafBprjMmqmknaSeu6h6zqueaKWfqMFursCnopqanJp6tMDAq6por5iWwW610mahmq5encGuuA%3D%3D